Tiptap的提及组件Mention同时添加多个实例的方法
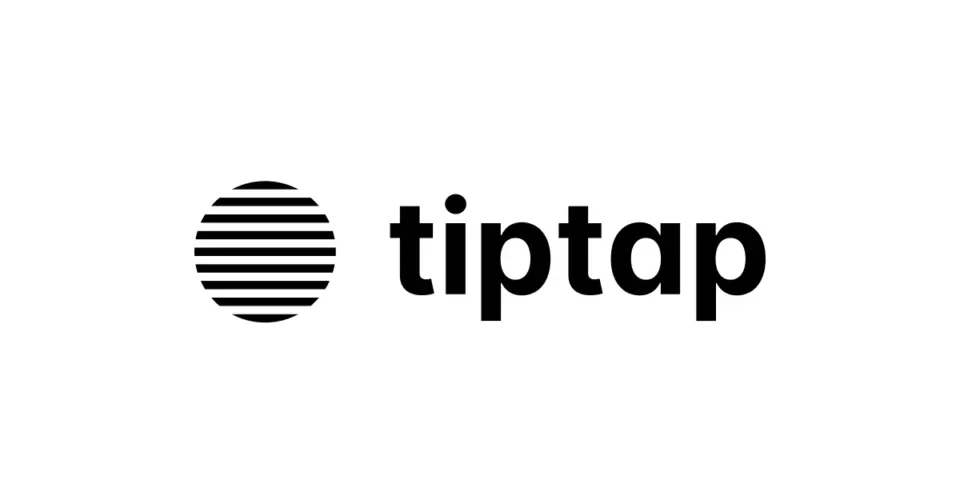
文章说明
我有使用多个提及符的使用场景,并且每一种提及符对应的数据集以及 UI 均不同,所以就找到了以下解决方案。
首先,我们需要按照 Tiptap 官方文档,安装 Tiptap ,我这里使用的是 Vue3,对其他前端框架一样起作用,只是相应的语法可能有些改变。
实现方式
Tiptap 安装
1 | npm install @tiptap/vue-3 @tiptap/pm @tiptap/starter-kit @tiptap/extension-mention |
创建 Editor 组件
1 | <template> |
在你的页面引入组件
1 | <template> |
引入 Mention 组件相关依赖
1 | npm install @tiptap/extension-mention |
创建 Mention 提及组件的 UI 组件 MentionList.vue
1 | <template> |
创建 Mention 提及组件的数据处理组件 suggestion.js
1 | import { VueRenderer } from '@tiptap/vue-3' |
在你的页面引入上述内容
1 | import Mention from '@tiptap/extension-mention' |
实现重点
实现 Mention 提及组件同时添加多个实例的方法就是,给每个 Mention 提及组件指定一个唯一的名称。
1 | Mention |
如上述代码所示,在 Mention.extend
中设置了 Mention 实例的 name
属性,如果你要创建多个,将他们的 name
属性区分好就可以了。
除了需要修改 name
属性以外,还需要修改的一处地方是 suggestion.js
文件中的 pluginKey
属性。
1 | import tippy from 'tippy.js' |
如上述代码所示,PluginKey
同样需要保持不同,这样就实现了 Tiptap 的提及组件 Mention 同时添加多个实例。
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 ProjectAn!
评论
你无需删除空行,直接评论以获取最佳展示效果